Fantastic Football-2D
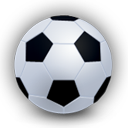
You may wounder about the tittle itself why i have suited this tittle, it is all for attracting . However, this article guides you to how to develop your own games in .NET Platform. if you have experience in windows application and web based application (ASP.Net), you just need to learn little bit more to develop game. By my experience, Unlike windows based and web application, XNA does not provide user interface in Compile time (Form Designing phase) Because game is fully graphical dependent.Here,you need somewhat mathematical knowledge in Trigonometric , Statistics and Game Logic.
Before starting, you have to prepare your system for game Development Environment by installing XNA game Studio.
What is XNA game Studio ?
XNA Game Studio and the XNA Framework are designed for cross-platform gaming scenarios with support for Windows Phone 7, Xbox 360, and Windows-based PCs. This allows you to target more platforms from the same code base. It also allows developers to focus game design for each platform on real device differences such as the device capabilities and experience, as opposed to writing with different frameworks for each targeted device.
- Download and Install XNA game studio from here
- Download Application File
- Download Project file
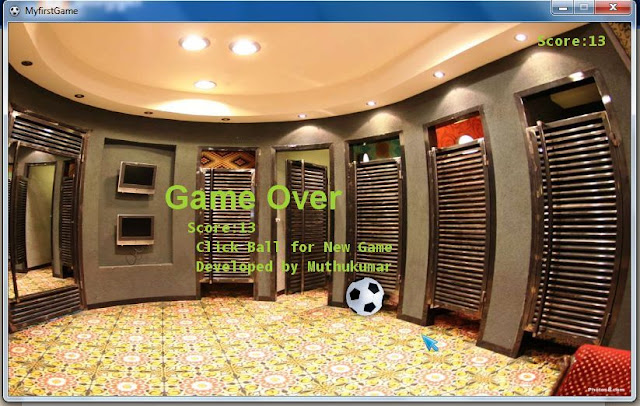
Part I - Basic Settings:
- As usual goto file--> New Project
- Expand visual C# Node, select XNA game studio and Name the Default Assemblies
After creating a new project, you'll be presented with the code view of your game.
Part II - View of code:
Some of the hard work has already been done for you. If you build and run your game now, the GraphicsDeviceManager will set up your screen size and render a blank screen. Your game will run and update all by itself. It's up to you to insert your own code to make the game more interesting.
Much of the code to start and run your game has already been written for you. You can insert your own code now.
These are the five methods allows you to code and run the game
- The Initialize method is where you can initialize any assets that do not require a GraphicsDevice to be initialized.
- The LoadContent method is where you load any necessary game assets such as models and textures.
- The UnloadContent method is where any game assets can be released. Generally, no extra code is required here, as assets will be released automatically when they are no longer needed.
- The Update loop is the best place to update your game logic: move objects around, take player input, decide the outcome of collisions between objects, and so on.
- The Draw loop is the best place to render all of your objects and backgrounds on the screen.
Part III - ADD Sprite
The next step is to add a graphic that can be drawn on the screen. Use a small graphics file, such as a small .bmp or .jpg file. Be creative—you can even make your own. You can even skip ahead a bit and make a sprite that "hides" parts that should not be seen (such as edges or corners) so that it looks even better.
- Make sure you can see the Solution Explorer for your project on the right side of the window. If you cannot see it, click the View menu, and then click Solution Explorer.
- Right-click the Content node, click Add, click Existing Item, and then browse to your graphic.
- If you can't see any files, make sure you change the Files of type selection box to read Texture Files.
- Click the graphic file, and then click Add.
Properties Window.
Like above Add Bed Room image file and football image to content folder.
Now Initialize these variables...
Texture2D mytex,bgr,mou; //these varible for background,football and Cursor
Vector2 sposition = Vector2.Zero;
Vector2 text = new Vector2(680, 10);
Vector2 gameover = new Vector2(200, 200);
Vector2 gameover1 = new Vector2(230, 250);
Vector2 spriteSpeed = new Vector2(0f, 10f);
Vector2 mpos = Vector2.Zero;
Vector2 origin;
Vector2 screenpos;
float RotationAngle;
GraphicsDevice device;
MouseState mouseStateCurrent;
SpriteFont fnt,gover;
Update "protected override void LoadContent() " method by adding...
device = graphics.GraphicsDevice;
spriteBatch = new SpriteBatch(GraphicsDevice);
mytex = Content.Load<Texture2D>("fb");
bgr = Content.Load <Texture2D>( "bg");
mou = Content.Load<Texture2D>("pon");
fnt = Content.Load<SpriteFont>("Arial");
gover = Content.Load<SpriteFont>("over");
Viewport viewport = graphics.GraphicsDevice.Viewport;
origin.X = mytex.Width / 2;
origin.Y = mytex.Height / 2;
screenpos.X = viewport.Width / 2;
screenpos.Y = viewport.Height / 2;
update "protected override void Draw(GameTime gameTime)" by adding..
int screenWidth;
int screenHeight;
graphics.GraphicsDevice.Clear(Color.CornflowerBlue);
// TODO: Add your drawing code here
spriteBatch.Begin();
screenWidth = device.PresentationParameters.BackBufferWidth;
screenHeight = device.PresentationParameters.BackBufferHeight;
Rectangle screenRectangle = new Rectangle(0,0, screenWidth, screenHeight);
spriteBatch.Draw(bgr, screenRectangle, Color.White);
spriteBatch.Draw(mytex, sposition,null,
Color.White,RotationAngle,origin,0.5f,SpriteEffects.FlipHorizontally,1f);
spriteBatch.Draw(mou,mpos,Color.White);
spriteBatch.DrawString(fnt, "Score:"+i.ToString(),text,Color.YellowGreen);
if(staus==true)
{
spriteBatch.DrawString(gover,"Game Over", gameover,Color.YellowGreen);
spriteBatch.DrawString(fnt, "Score:" + i.ToString()+"\n Click Ball for
New Game\n Developed by Muthukumar", gameover1, Color.YellowGreen);
spriteSpeed = new Vector2(0f, 0f);
}
spriteBatch.End();
base.Draw(gameTime);
Update " protected override void Update(GameTime gameTime)" method by..
This is the right place to show your mathematical talents and game logic...
mpos.X = mouseStateCurrent.X;
mpos.Y = mouseStateCurrent.Y;
RotationAngle +=(float) gameTime.ElapsedGameTime.TotalSeconds*5;
float circle = MathHelper.Pi * 2;
RotationAngle = RotationAngle % circle;
int MaxX =
graphics.GraphicsDevice.Viewport.Width - mytex.Width;
int MinX = 0;
int MaxY =
graphics.GraphicsDevice.Viewport.Height - mytex.Height;
int MinY = 0;
mouseStateCurrent = Mouse.GetState();
if (mouseStateCurrent.LeftButton == ButtonState.Pressed)
{
if (staus == true)
{
staus = false;
i = 0;
}
if (Math.Abs(sposition.Y - mouseStateCurrent.Y) <50 &&
Math.Abs(sposition.X - mouseStateCurrent.X) < 50)
{
spriteSpeed = new Vector2(mouseStateCurrent.Y-100,
mouseStateCurrent.X-100);
sposition -=
spriteSpeed * (float)gameTime.ElapsedGameTime.TotalSeconds * 1;
i++;
}
}
else
{
sposition -=
spriteSpeed * (float)gameTime.ElapsedGameTime.TotalSeconds * 2;
}
// Check for bounce.
if (sposition.X > MaxX+50)
{
spriteSpeed.X *= -1;
sposition.X = MaxX;
i+=5;
}
else if (sposition.X < MinX)
{
spriteSpeed.X *= -1;
sposition.X = MinX;
i++;
}
if (sposition.Y > MaxY+75)
{
spriteSpeed.Y *= -1;
sposition.Y = MaxY;
i++;
staus = true;
}
else if (sposition.Y < MinY)
{
spriteSpeed.Y *= -1;
sposition.Y = MinY;
i++;
}
base.Update(gameTime);
PART V:
- Build and run your game.
Preview of game:
Enjoy the game..............
Post your Valuable Comments.........